# QField Plugins workshop ### FOSS4G 2024 - Belรฉm, Brasil
--- ## Who we are --v-- --v-- ## Who you are
--v-- ## AGENDA 1. Why plugins for QField? 2. Types of QField plugins 3. Intro to QML ๐งโ๐ป 4. Developing QField plugins ๐งโ๐ป 5. Plugin demo: OSRM routing 6. Recreating the Weather Forecast plugin! ๐งโ๐ป 7. Plugin showcase 8. Resources -----------
๐งโ๐ป: Practice!
--- ## Why plugins for QField? --- ## TYPES OF QFIELD PLUGINS PROJECT PLUGINS | APPLICATION PLUGINS ----------------|-------------------- Shared alongside QField project | Shared via URL (ZIP file) Single plugin | Multiple plugins Installation not required | Required installation Lasts project session | Lasts QField session --- ## INTRO TO QML ๐งโ๐ป +
Q
t
M
odeling
L
anguage, declarative, design-oriented --v-- ## INTRO TO QML ๐งโ๐ป +
Q
t
M
odeling
L
anguage, declarative, design-oriented + **Elements (aka types)** --v-- ### \.\./Elements (aka types) ```Item, Rectangle, Text, TextInput, MouseArea, Dialog, ...``` ---- ``` qml Text { ... } ``` --v-- ### \.\./Elements (aka types) ```Item, Rectangle, Text, TextInput, MouseArea, Dialog, ...``` ---- ``` qml Rectangle { ... Text { ... } } ``` --v-- ### \.\./Elements (aka types) ```Item, Rectangle, Text, TextInput, MouseArea, Dialog, ...``` ---- ``` qml Item { ... Text { ... } } ``` --v-- ## INTRO TO QML ๐งโ๐ป +
Q
t
M
odeling
L
anguage, declarative, design-oriented + Elements (aka types) + **Attributes: Properties, Signals, Handlers and Methods** --v-- ### \.\./Attributes: Properties, Signals, Handlers and Methods
``` qml Rectangle { ... MouseArea { id: myArea ... } } ```
--v-- ### \.\./Attributes: Properties, Signals, Handlers and Methods
``` qml Rectangle { ... MouseArea { id: myArea color: "green" anchors.fill: parent } } ```
--v-- ### \.\./Attributes: Properties, Signals, Handlers and Methods
``` qml Rectangle { ... MouseArea { id: myArea color: "green" anchors.fill: parent onClicked: ... } } ```
--v-- ### \.\./Attributes: Properties, Signals, Handlers and Methods
``` qml Rectangle { ... MouseArea { id: myArea color: "green" anchors.fill: parent onClicked: ... function myCustomMethod() { ... return ...; } } } ```
--v-- ### \.\./Attributes: Properties, Signals, Handlers and Methods
``` qml Rectangle { ... MouseArea { id: myArea color: "green" anchors.fill: parent // Property binding! onClicked: ... function myCustomMethod() { ... return ...; } } } ```
--v-- ## INTRO TO QML ๐งโ๐ป +
Q
t
M
odeling
L
anguage, declarative, design-oriented + Elements (aka types) + Attributes: Properties, Signals, Handlers and Methods + **QML Resources** --v-- ### \.\./QML Resources
Introduction to Qt/QML, by KDAB: [YouTube Playlist](https://www.youtube.com/watch?v=JxyTkXLbcV4&list=PL6CJYn40gN6hdNC1IGQZfVI707dh9DPRc&index=2) QML Reference: https://doc.qt.io/qt-5/qmlreference.html QML Tutorial: https://doc.qt.io/qt-5/qml-tutorial.html --v-- ## INTRO TO QML ๐งโ๐ป +
Q
t
M
odeling
L
anguage, declarative, design-oriented + Elements (aka types) + Attributes: Properties, Signals, Handlers and Methods + Resources + **Hello world (QML)!** ๐ --v-- ### \.\./Hello world (QML) ๐ ``` qml import QtQuick 2.0 Text { text: "Hello world!" } ``` --v-- ## INTRO TO QML ๐งโ๐ป +
Q
t
M
odeling
L
anguage, declarative, design-oriented + Elements (aka types) + Attributes: Properties, Signals, Handlers and Methods + Resources + Hello world (QML)! ๐ + **Exercise online!** ๐งโ๐ป --v-- ## \.\./Exercise online! ๐งโ๐ป
Go to: https://qmlweb.github.io --- ## Developing QField plugins ๐งโ๐ป + **QField GUI** --v-- ## \.\./QField GUI
Dashboard
| Main menu | Search bar | Plugins toolbar | Positioning | Canvas actions
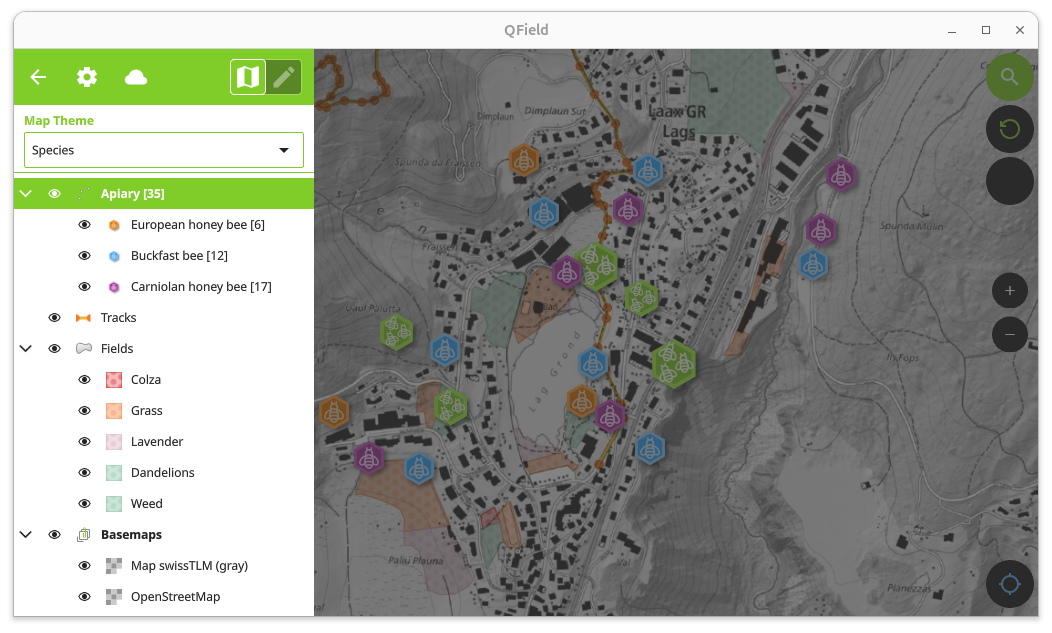 --v-- ## \.\./QField GUI
Dashboard |
Main menu
| Search bar | Plugins toolbar | Positioning | Canvas actions
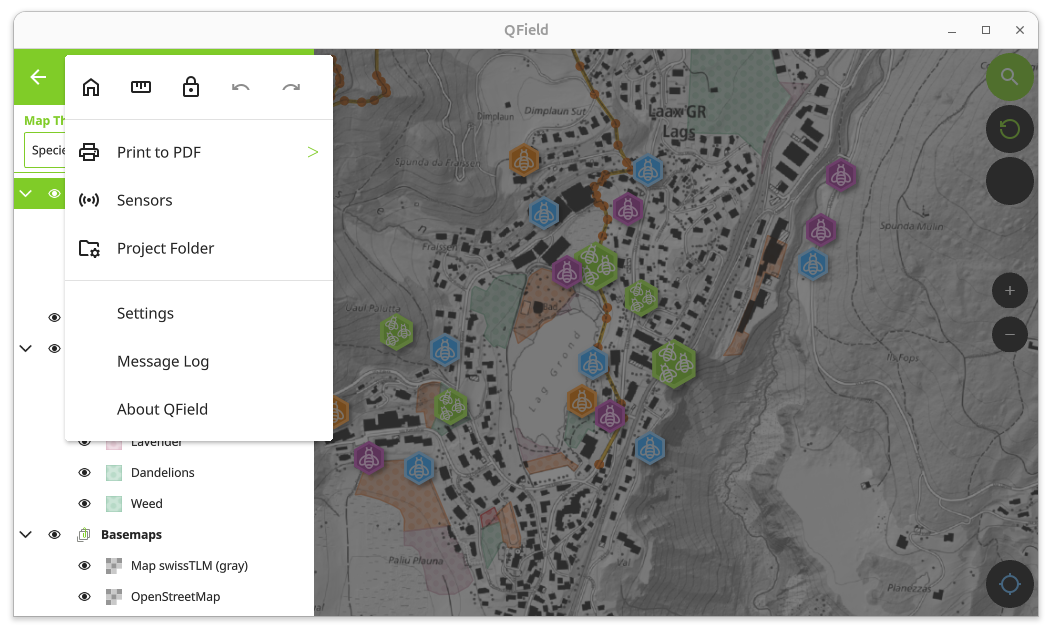 --v-- ## \.\./QField GUI
Dashboard | Main menu |
Search bar
| Plugins toolbar | Positioning | Canvas actions
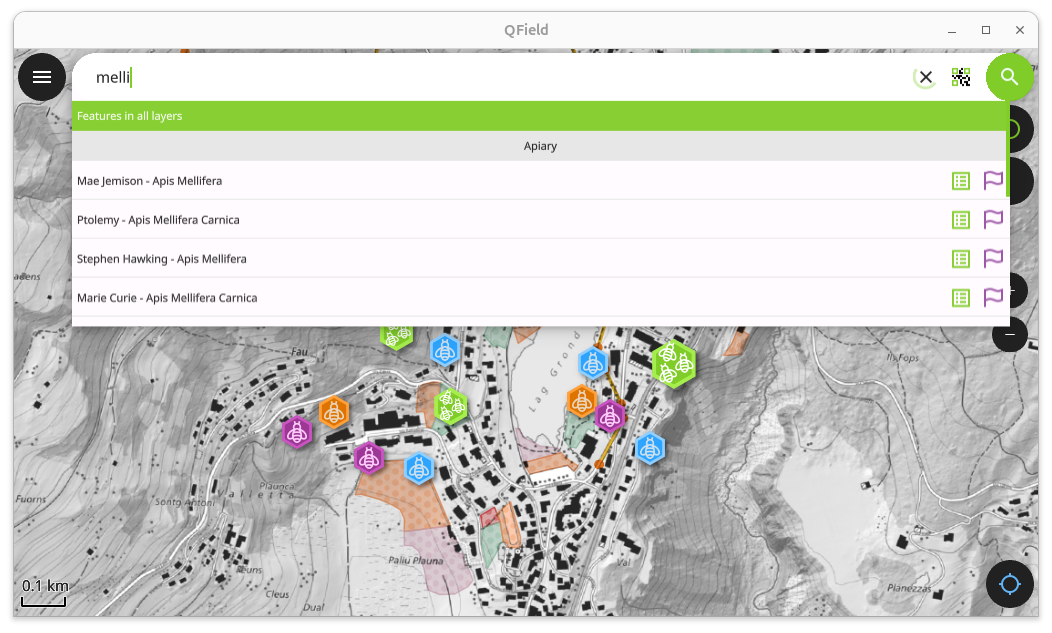 --v-- ## \.\./QField GUI
Dashboard | Main menu | Search bar |
Plugins toolbar
| Positioning | Canvas actions
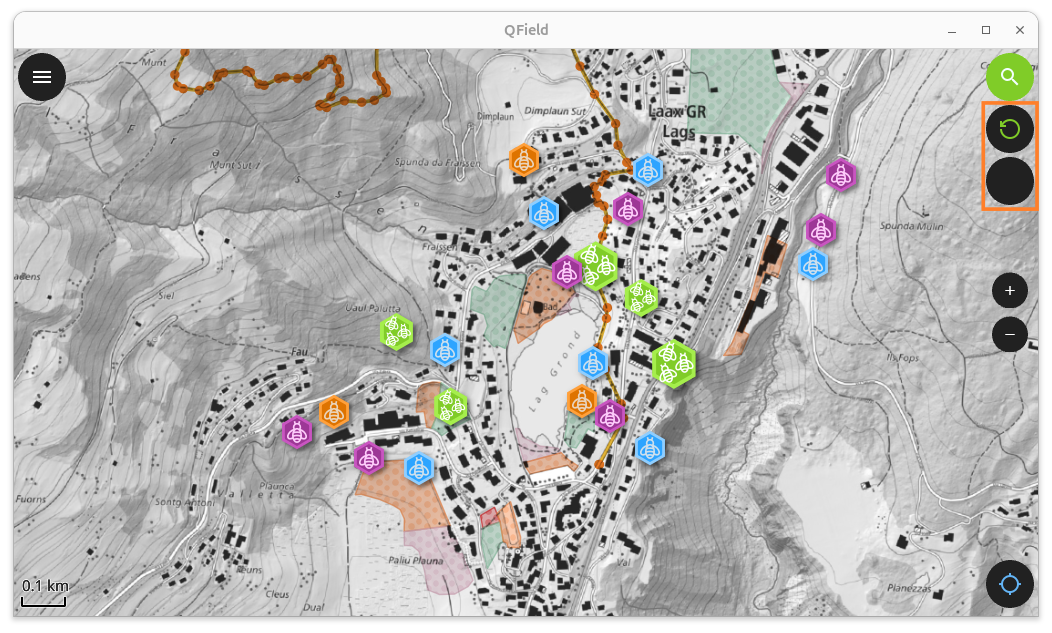 --v-- ## \.\./QField GUI
Dashboard | Main menu | Search bar | Plugins toolbar |
Positioning
| Canvas actions
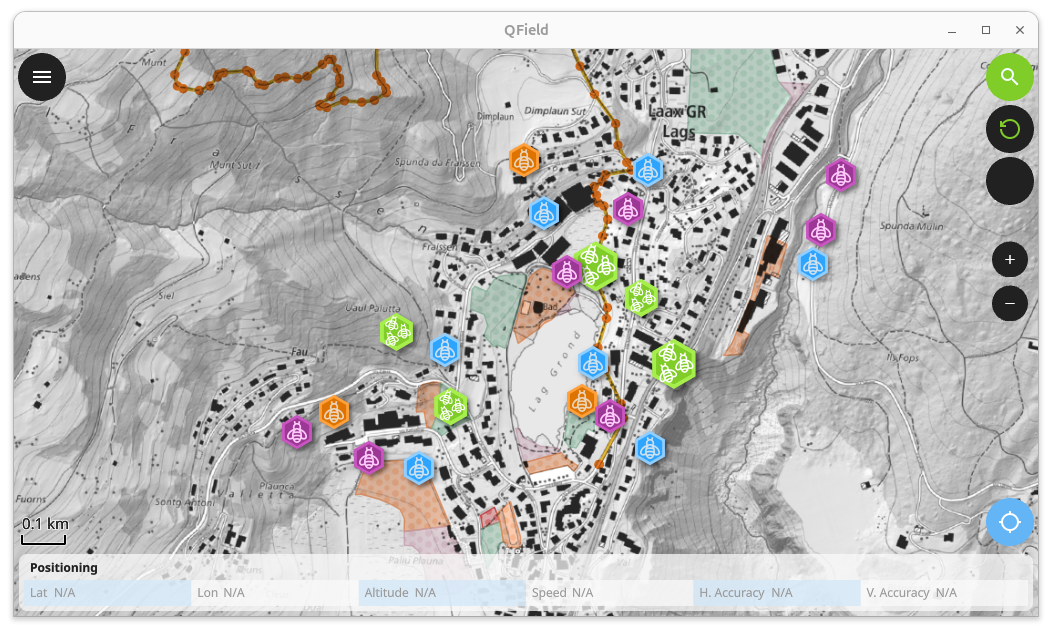 --v-- ## \.\./QField GUI
Dashboard | Main menu | Search bar | Plugins toolbar | Positioning |
Canvas actions
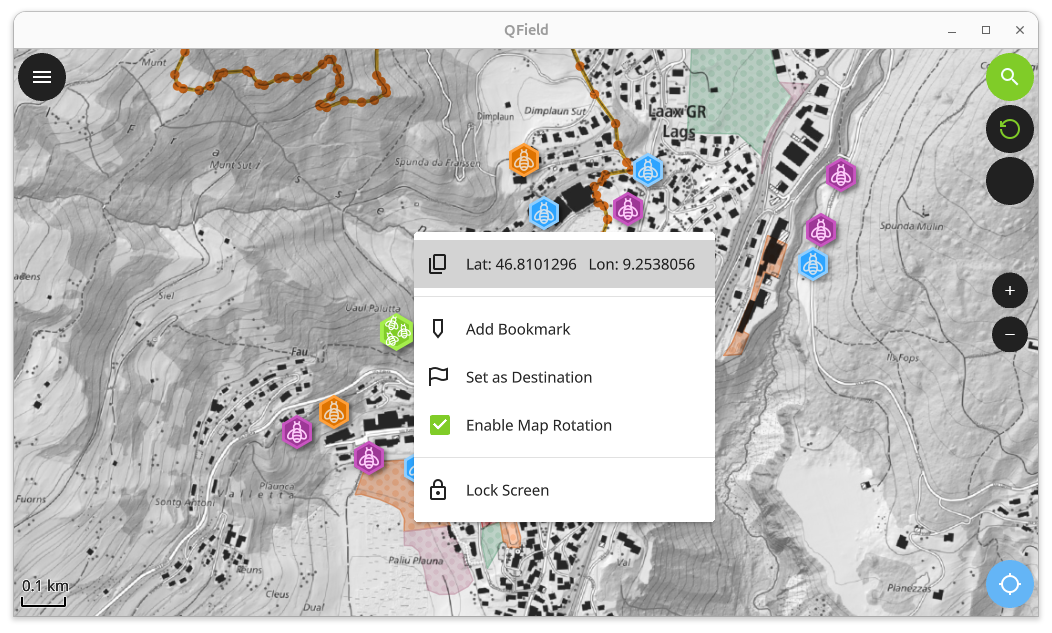 --v-- ## Developing QField plugins ๐งโ๐ป + QField GUI + **QField API** ๐ --v-- ## \.\./QField API ๐ ####
Root objects
| iface | Theme | QField GUI types | Utils
-------- ```iface``` ```qgisProject``` ```settings``` ```clipboardManager``` ```...``` --------
_See [qgismobileapp.cpp](https://github.com/opengisch/QField/blob/master/src/core/qgismobileapp.cpp#L574-L591) for details._
--v-- ## \.\./QField API ๐ ####
Root objects |
iface
| Theme | QField GUI types | Utils
-------- ```iface.mainWindow()``` ```iface.mapCanvas()``` ```iface.findItemByObjectName("...")``` ```iface.logMessage("...")``` -------- ```iface.addItemToPluginsToolbar()``` ```iface.addItemToMainMenuActionsToolbar()``` ```iface.addItemToCanvasActionsToolbar()``` --------
_See [appinterface.h](https://github.com/opengisch/QField/blob/master/src/core/appinterface.h) for details._
--v-- ## \.\./QField API ๐ ####
Root objects | iface |
Theme
| QField GUI types | Utils
-------- ``` qml Theme.mainColor // #80cc28 Theme.mainTextColor Theme.mainBackgroundColor Theme.darkGray Theme.defaultFont Theme.getThemeVectorIcon("...") ``` --------
_See [Theme.qml](https://github.com/opengisch/QField/blob/master/src/qml/imports/Theme/Theme.qml) for details._
--v-- ## \.\./QField API ๐ ####
Root objects | iface | Theme |
QField GUI types
| Utils
-------- ```QfButton``` ```QfToolButton``` ```QfTextField``` ```QfSwitch``` ```QfComboBox``` ```...``` --------
_See [src/qml/imports/Theme/*](https://github.com/opengisch/QField/blob/master/src/qml/imports/Theme/Theme.qml) for details._
--v-- ## \.\./QField API ๐ ####
Root objects | iface | Theme | QField GUI types |
Utils
-------- ```LayerUtils``` ```GeometryUtils``` ```FeatureUtils``` ```CoordinateReferenceSystemUtils``` ```SnappingUtils``` ```...``` --------
_See [src/core/utils/*](https://github.com/opengisch/QField/tree/master/src/core/utils) for details._
--v-- ## Developing QField plugins ๐งโ๐ป + QField GUI + QField API + **"Hello world"** ๐ ``` qml import QtQuick Item { Component.onCompleted: { iface.mainWindow().displayToast('Hello world!') } } ``` --v-- ## Developing QField plugins ๐งโ๐ป + QField GUI + QField API + "Hello world" + **Debugging** ๐ ```iface.logMessage("")``` ```mainWindow.displayToast("")``` ```console.log("")``` ? --v-- ## Developing QField plugins ๐งโ๐ป + QField GUI + QField API + "Hello world" + Debugging + **QField template plugin** ๐งโ๐ป https://github.com/opengisch/qfield-template-plugin --- ## OSRM routing plugin demo
--- ## Weather Forecast plugin ๐งโ๐ป Let's recreate this plugin! What we need: + Button to fetch a weather forecast and show an info dialog + Our location + API call: + _XmlHttpRequest_ to do an API call and fetch the forecast + Parse the request's response + Set description (and temperature) QML properties --- ## Plugin showcase Snap! 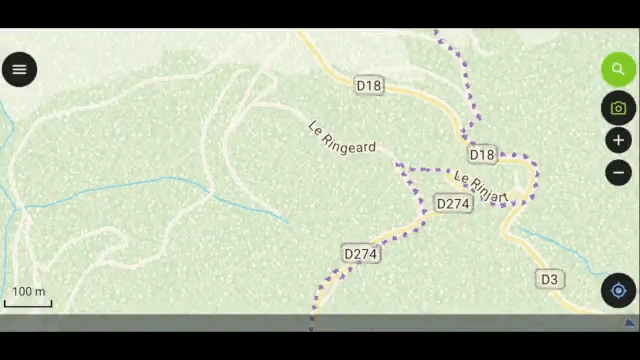 --v-- ## Plugin showcase Nominatim locator plugin https://github.com/opengisch/qfield-nominatim-locator --v-- ## Plugin showcase LiveField plugin
--- ## Resources QML Reference: https://doc.qt.io/qt-5/qmlreference.html Introduction to Qt/QML, by KDAB ([YouTube Playlist](https://www.youtube.com/watch?v=JxyTkXLbcV4&list=PL6CJYn40gN6hdNC1IGQZfVI707dh9DPRc&index=2)) QML online editor: https://qmlweb.github.io QML advanced online editor: https://patrickelectric.work/qmlonline/ QML Tutorial: https://doc.qt.io/qt-5/qml-tutorial.html QField plugins: https://github.com/topics/qfield-plugin Plugin icons: https://fonts.google.com/icons --- 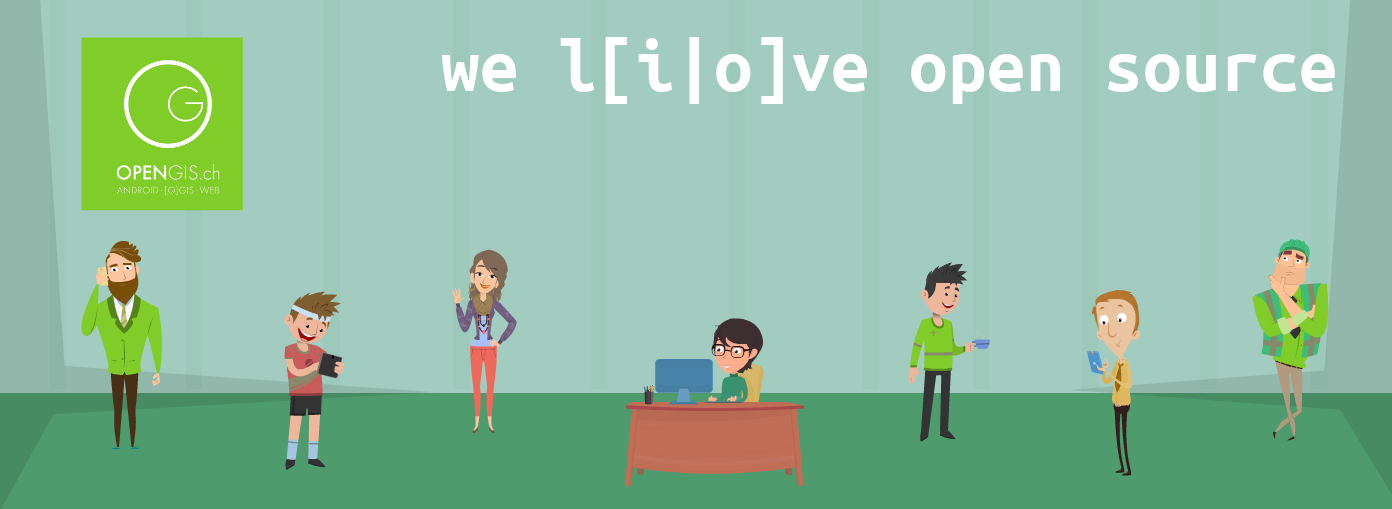 Thanks, and happy coding!